Create your own helper functions on Laravel4
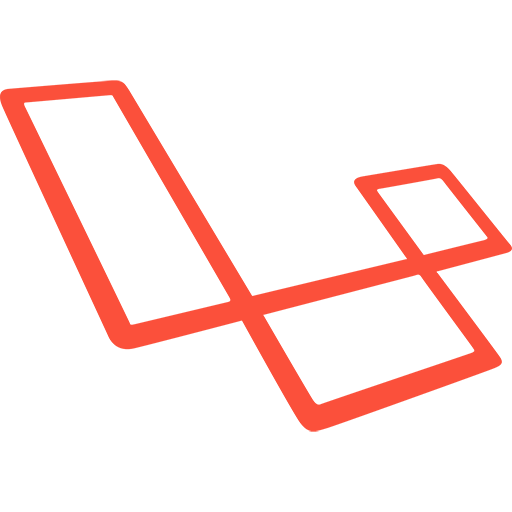
There are some common functions that I need to use on almost every controller but I don't want to write it over and over again in each controller. For this purpose, you create helpers, tell Laravel to look for it and then use it whenever you want, sounds cool right?
Showing it with an example will be better i guess:
Let's say you need to parse a specific kind of string in controllers (maybe you are using slugs for URLs?), and you don't want to write that parsing function again in every controller, here is the solution:
Create a file app/helper/URLHelper.php
and then put something like this in that file:
<?php
class URLHelper {
public static function parseURL($url)
{
$id = explode('-', $slug);
$id = $id[0];
return $id;
}
}
and to make Laravel aware of this file, add it to the composer.json
's autoload
part:
...
"autoload": {
"classmap": [
...
"app/helpers",
]
},
...
don't forget to reload the autoload with composer:
$ composer dump-autoload
All set and ready to go, now you can use this method in your controller like this:
$id = URLHelper::parseURL($url);